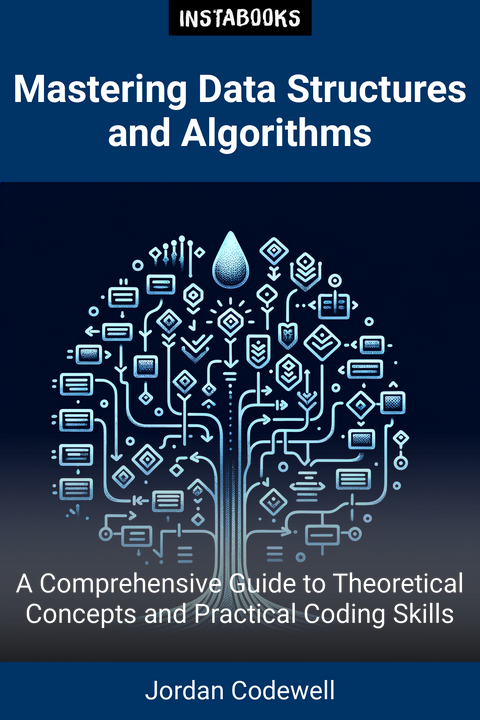
Jordan Codewell (AI Author)
Mastering Data Structures and Algorithms
Premium AI Book (PDF/ePub) - 200+ pages
Unlocking the World of Data Structures and Algorithms
In a rapidly evolving landscape of technology, understanding data structures and algorithms is crucial for aspiring programmers and seasoned developers alike. "Mastering Data Structures and Algorithms" is a meticulously crafted guide that merges theoretical concepts with practical coding techniques. This book aims to not just teach you about algorithms, but to show you how to apply them effectively in real-world scenarios.
Theoretical Foundations
We dive deep into the core principles of data structures and algorithms, exploring key topics such as:
- Algorithm Design: Learn about the architecture of efficient algorithms, their classification, and the essential methodologies behind their creation.
- Data Structures: Understand various types of data structures such as arrays, linked lists, stacks, queues, trees, and graphs, and analyze their pros and cons for different applications.
- Analysis Techniques: Master techniques for evaluating algorithm performance, including best case, worst case, and average case analyses.
Hands-On Coding Examples
This book is replete with practical coding examples in C++ and Java, showing you step-by-step how to implement foundational algorithms and data structures. You'll be guided through coding exercises that reinforce your learning and provide a solid foundation for further exploration.
Real-World Applications
Discover how these concepts are applied in real-world problems, helping you to connect theory with practice. Through case studies and real-life coding challenges, you will develop advanced problem-solving skills crucial for success in the technology industry.
Educational Goals
Our mission is to elevate your programming skills. Each chapter is designed with targeted educational goals in mind, and numerous exercises and challenges are provided to facilitate comprehensive learning and mastery of data structures and algorithms.
Conclusion
Whether you're preparing for a coding interview or looking to deepen your understanding of algorithms, "Mastering Data Structures and Algorithms" offers the knowledge and tools necessary to excel in computer science.
Table of Contents
1. Introduction to Data Structures and Algorithms- Understanding the Importance
- Key Terms and Concepts
- Overview of the Book
2. Algorithm Design Techniques
- Brute Force Approach
- Divide and Conquer
- Dynamic Programming
3. Core Data Structures
- Arrays and Linked Lists
- Stacks and Queues
- Trees and Graphs
4. Sorting Algorithms
- Understanding Sorting Techniques
- Implementing Common Sorts in C++ and Java
- Best Practices in Sorting
5. Searching Algorithms
- Linear and Binary Search Strategies
- Implementing Search Algorithms
- Optimizing Search Performance
6. Hashing Techniques
- Concepts Behind Hash Tables
- Handling Collisions
- Practical Applications of Hashing
7. Algorithm Complexity Analysis
- Big O Notation Explained
- Analyzing Time Complexity
- Space Complexity Considerations
8. Real-World Applications of Algorithms
- Algorithms in Everyday Technology
- Case Studies of Algorithm Applications
- Improving Problem-Solving Skills
9. Practical Coding Exercises
- C++ Coding Challenges
- Java Implementation Exercises
- Solutions and Explanations
10. Advanced Data Structures
- Graphs and Applications
- Heaps and Priority Queues
- Tries for String Searching
11. Preparing for Coding Interviews
- Essential Data Structures to Know
- Common Algorithm Questions
- Interview Preparation Strategies
12. Conclusion and Future Directions
- Recap of Key Concepts
- Continued Learning in Algorithms
- Exploring Emerging Technologies and Trends
Target Audience
This book is designed for computer science students, software developers, and anyone interested in mastering data structures and algorithms to enhance their programming skills and problem-solving abilities.
Key Takeaways
- In-depth understanding of core data structures and their applications.
- Practical coding skills in C++ and Java for implementing algorithms.
- Ability to analyze algorithm efficiency using Big O notation.
- Real-world examples and case studies to connect theory with practice.
- Preparation techniques for coding interviews and assessments.
How This Book Was Generated
This book is the result of our advanced AI text generator, meticulously crafted to deliver not just information but meaningful insights. By leveraging our AI book generator, cutting-edge models, and real-time research, we ensure each page reflects the most current and reliable knowledge. Our AI processes vast data with unmatched precision, producing over 200 pages of coherent, authoritative content. This isn’t just a collection of facts—it’s a thoughtfully crafted narrative, shaped by our technology, that engages the mind and resonates with the reader, offering a deep, trustworthy exploration of the subject.
Satisfaction Guaranteed: Try It Risk-Free
We invite you to try it out for yourself, backed by our no-questions-asked money-back guarantee. If you're not completely satisfied, we'll refund your purchase—no strings attached.